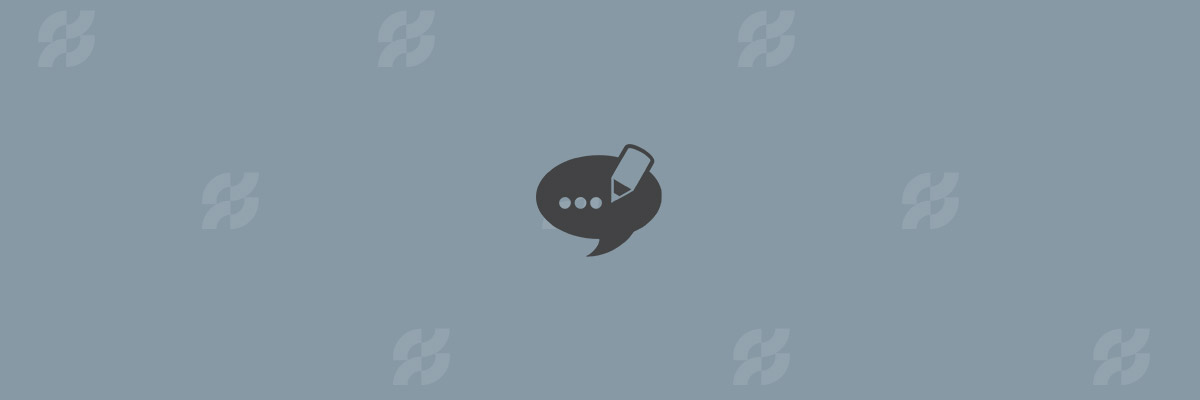
Welcome to Ferguson’s Developer Portal!
Introduction
In this blog post we are going to go over OAuth 2.0 Authorization Framework, delve into the easiest Authorization Grant called Client Credentials, and give some useful information about how it works.
Quick explanation of OAuth 2.0
When trying to explain to someone what OAuth 2.0 is, I always think of the phrase “Access Delegation”. It’s a means by which you, the end user or resource owner, can get a token and then share or give that token to a client to access resources on your behalf without giving that client your password. I think Wikipedia’s first paragraph sums up OAuth nicely:
“OAuth is an open standard for access delegation, commonly used as a way for Internet users to grant websites or applications access to their information on other websites but without giving them the passwords.”
OAuth 2.0 Roles
You can’t talk about delegating access without understanding who is giving access to what. OAuth refers to these as “Roles” and here they are shortened for brevity:
- Resource Owner: The entity who is granting access to a protected resource.
- Resource Server: The thing that is protected and takes access tokens. In our case, the REST API.
- Client: The application you don’t want to give your password too but needs to access the Resource Server.
- Authorization Server: The server that issues an access token once you have authenticated successfully as the Resource Owner.
OAuth 2.0 Grant types
When working with OAuth 2.0 “Access Delegation” patterns, you will commonly hear them being referenced as “Grants” as you’re “Granting” access or “Authorizing” a client which you’d rather not give your password too. You will also hear grants referenced as “Authorization Grants”.
The OAuth 2.0 SPEC defines 4 unique Grant Types which are called:
- Client Credentials
- Resource Owner Password Credentials (ROPC or Password Grant Type)
- Implicit
- Authorization Code
Each one of these fits a specific use case and if you’re curious which OAuth 2.0 Grant Type should be used for your particular situation, take a look at Alex Bilbie’s website as he’s got a snazzy decision workflow diagram towards the bottom of the page. We will eventually cover all of these OAuth2 grants here on our blog.
Client Credentials
The first grant type we’re going to discuss is Client Credentials. Client Credentials is only used in situations where you are talking between machines or backend service accounts who don’t use an interactive session. Because of this, Client Credentials grant type is one of the easiest grant types to understand as there’s not much to it. You can break it down to two steps really.
- First step is getting an access token by giving the Authorization Server proof that it is really you and when your token expires, say in one hour, getting another one.
- Second step is taking that access token and giving it to the Resource Server when you need something like making an API call.
And that is pretty much it. Because this grant type is used by machine accounts, it does not get a refresh token just an access token.
Getting an access token from Ferguson
The first step to making an API call to Ferguson is to get an access token but before that, you will need to be registered with our Developer Portal, have created a Developer App, and gotten your Credentials.
The login link is in the upper righthand corner of the page.
Once logged in, go to your “My Apps” page and select the button to the far right called “Register New App”.
The “Register New App” located on the right of the picture above and outlined in RED.
Now you need to come up with an App name and select the API Product that is right for you. For the purposes of this blog post, I am going to use the “HelloWorld” Product.
Once you’re all filled out, then click the “Create App” button at the bottom of the page.
Now you will have an App Key and App Secret which get exchanged for an access token and allow access specifically to the HelloWorld API Product that we selected earlier.
Here you can see my Key = FqCHNSblIS9Agf7JLpn2KGBLOVLBwJUi and my Secret = rx2KpqAjpT8NB7yt
NOTE: We’re going to go over in detail the Client Credentials Grant Flow but your software code shouldn’t be doing this by hand! Make sure to hit the internet to find an OAuth2 library for your specific application. OAuth.net has a nice listing of OAuth2 libraries per language.
UML Sequence diagram of Client Credentials Grant Flow
Let’s look at this flow using an UML sequence diagram to understand how Ferguson exchanges credentials (Key & Secret) for fetching an access token. While Ferguson is using the preferred method of HTTP Basic authentication there are other ways of authenticating to get an access token such as client_id and client_secret in the request-body but they all need to be encoded using a Content-Type of “application/x-www-form-urlencoded”.
- We are going to make an API call to the OAuth2 token endpoint using our credentials from the developer portal in the form of a Basic authentication header that’s base64 encoded with padding.
- Take our Key & Secret and base64 encode them like so:
base64 encode with padding (<KEY> + “:” + <SECRET>)
FqCHNSblIS9Agf7JLpn2KGBLOVLBwJUi: rx2KpqAjpT8NB7yt
Which becomes: RnFDSE5TYmxJUzlBZ2Y3SkxwbjJLR0JMT1ZMQndKVWk6cngyS3BxQWpwVDhOQjd5dA==
- Now, take the base64 encoded value and use it as a Basic Authorization HTTP request header, set our Content-Type and add a form parameter of grant_type=client_credentials.
- If you are following along and using curl, your request might look like this:
curl -X POST \ https://sandboxapi2.ferguson.com/oauth2/token \ -H 'Authorization: Basic RnFDSE5TYmxJUzlBZ2Y3SkxwbjJLR0JMT1ZMQndKVWk6cngyS3BxQWpwVDhOQjd5dA==' \ -H 'Content-Type: application/x-www-form-urlencoded' \ -d 'grant_type=client_credentials'
- Take our Key & Secret and base64 encode them like so:
- Once your API call hits the API Gateway, it decodes your Basic Authorization header and makes sure your creds are still valid and enabled.
- If your credentials are still valid, it issues a JSON payload back to you with an access token and expires_in key/value pair. Typically access tokens expire after an hour and this is why it is critical that you use a library to manage your OAuth2 credentials. If your credentials weren’t valid, you’d get back a 401 Unauthorized response.
Using your access token to make a “HelloWorld” REST API call
Now that we have explained the entire flow of how you get an access token using Client Credentials Grant Type, let’s use it to call our API!
Just like getting the access token, we have divided the REST API call into multiple steps.
- We are going to craft our request payload with just an Authorization header that’s set using our access token and our token type of “Bearer”. There are a number of types of tokens but the OAuth2 RFC6750 specifically created a Bearer Token Usage document which defines the proper usage of Bearer tokens within the OAuth2 Authorization Framework. There are other types of Bearer tokens like JSON Web Tokens (JWTs) and OpenID Connect (OIDC) but we won’t get into those types of Bearer tokens in this blog post.
Our curl command would look like the following:curl -H 'Authorization: Bearer Fogs1kEE7lvoTGUkX7q55v3wfQBt' \ https://sandboxapi2.ferguson.com/v1/hello
Once the HTTPS request hits Ferguson’s API Gateway, its’ Bearer token is verified. If it is not valid, the API Gateway throws back a 401 Unauthorized response.
- Once the API Gateway has validated the Bearer token, it then checks other policies like quotas, spike arrests and IP address ACLs. If there had been any query string parameters or a request body, it would have done some level of validation on that too before finally sending it to the backend service’s API endpoint.
- The backend service responds:
- The backend service response initially hits Ferguson’s API Gateway where the gateway itself can do some level of validation or transformation on the backend’s response (maybe remove some response headers? Maybe change an XML response back to JSON?)
- Once the API Gateway is done with the response from the backend, it then forwards it to the API Consumer that made the initial API call.
Wrapping up OAuth2 Client Credentials Grant Type
While this might feel like a long blog post, we did cover a lot of information. We touched on OAuth2 being a framework for delegating authorization, the OAuth2 roles defined by the framework, and 4 authorization Grant Types with a super simple example of a Client Credentials grant with UML sequence diagrams and curl commands. We will go over the other 3 authorization Grant Types in future blog posts so stay tuned!